If Else Statement In Java
In this page, we will learn If Else Statement In Java, What is If Else Statement In Java?, If Statement in Java, If statement systax in java, If statement Example in Java, If Else Statement in Java, IF Else Example In Java, If Else If ladder Statement In Java, Nested if statement Statement In Java.
What is If Else Statement In Java?
The java “If statement” is employed to check the condition. It is a boolean condition checker : true or false. There are different types of if statement in Java.
- if statement
- if else statement
- if else if ladder
- nested if statement
If Statement in Java :-
The Java “If statement” tests the condition. It executes the “If block” if the condition is true.
If statement systax in java:
if( condition ) {
// code to be executed
}
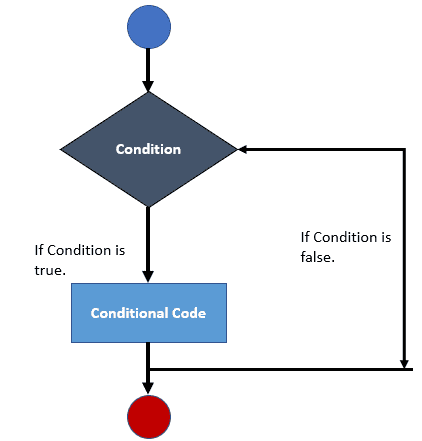
If statement Example in Java :-
import java.io.*;
public class Main {
public static void main(String args[]){
int age = 20;
if(age > 18) {
System.out.println("Over 18");
}
}
}
Output:
Over 18
If Else Statement in Java :-
The Java “If else statement” also tests the condition. It executes the “If block” if the condition is true otherwise else block is executed.
If else statement systax in Java :-
if( condition ) {
// code to be executed
}
else{
// code to be executed
}
IF Else Example In Java:-
import java.io.*;
public class Main {
public static void main(String args[]){
int age = 16;
if(age > 18) {
System.out.println("Over 18");
}
else{
System.out.println("Under 18");
}
}
}
Output:
Under 18
If Else If ladder Statement In Java:-
The “If else if ladder statement” executes one condition from multiple statements.
If else if ladder statement systax in Java :-
if( condition ) {
// code to be executed
}
else if( condition ) {
// code to be executed
}
else {
// code to be executed
}
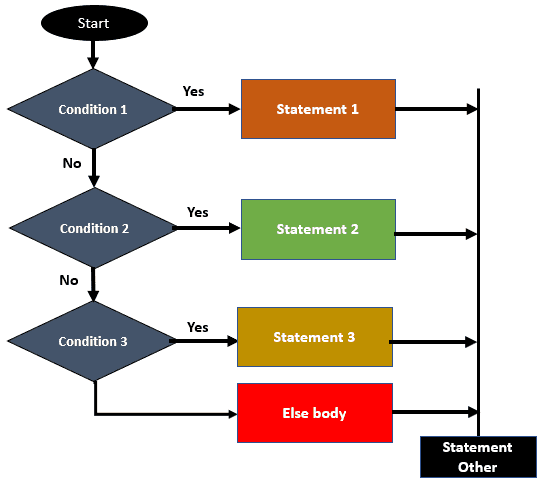
If else if ladder statement Example In Java:-
import java.io.*;
public class Main {
public static void main(String args[]){
int age = 18;
if(age > 18) {
System.out.println("Over 18");
}
if(age == 18) {
System.out.println("Just 18");
}
else{
System.out.println("Under 18");
}
}
}
Output:
Just 18
Nested if statement Statement In Java:-
The “Nested if statement” represents the “If block” within another “If block.” Here, the inner “If block” condition executes only when outer “If block” condition is true.
Nested if statement systax in Java :-
if( condition ) {
if( condition ) {
// code to be executed
}
}
else {
// code to be executed
}
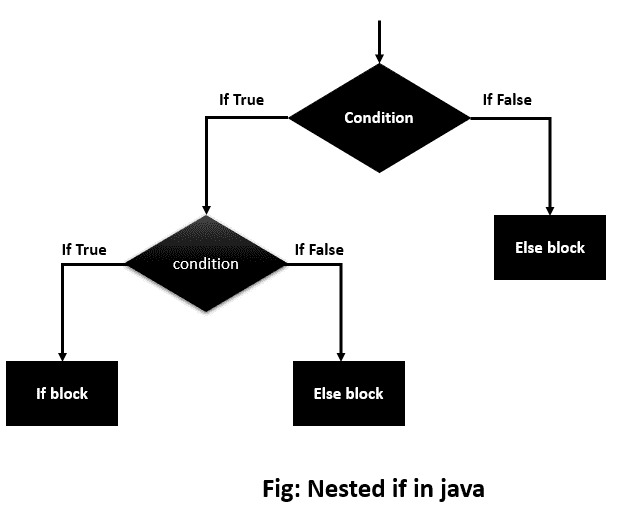
Nested if statement Example In Java:-
import java.io.*;
public class Main {
public static void main(String args[]){
int age = 19;
if(age > 18) {
if(age == 19) {
System.out.println("Just 19");
}
}
else{
System.out.println("Under 18");
}
}
}
Output:
Just 19