C Programming Tutorial
In our tutorial, you will learn the fundamentals of C programming language and progress to more advanced concepts. Here's what you can expect to learn:
- Basics of C Programming: We will cover the basics of C programming language, including data types, variables, constants, operators, control statements, and loops.
- Functions: You will learn how to create and use functions in C programming language, including how to pass parameters and return values.
- Arrays and Pointers: We will cover arrays and pointers in C programming language, including how to manipulate arrays and pointers, and how to use them in functions.
- Structures and Unions: We will explore structures and unions in C programming language, including how to create and use structures and unions, and how to pass them to functions.
- File Input/Output: We will cover file input/output operations in C programming language, including how to read and write data to and from files.
- Dynamic Memory Allocation: You will learn how to dynamically allocate memory in C programming language, including how to allocate and free memory using malloc(), calloc(), and realloc().
- Advanced Topics: Finally, we will cover some advanced topics in C programming language, including pointers to functions, bit manipulation, recurtion and preprocessor directives.
By the end of our c programing tutorial, you will have a thorough understanding of the C programming language and be able to write efficient and optimized C programs.
Why Our Tutorial is the best?
Our C programming tutorial is the best because:
- We provide a step-by-step guide for learning C programming.
- We provide real-life examples and scenarios for better understanding.
- Our tutorial is designed for beginners and advanced learners.
- We provide tips and tricks for writing efficient and optimized code in C programming.
- We cover all the important topics in C programming that are required to become an expert in it.
Let's gather some knowledge about c programming language, before we start.
What is C Programming Language?
C programming language is a high-level programming language that was initially developed by Dennis Ritchie in 1972 at Bell Labs. It was created as an extension of the popular B programming language and was designed to be a general-purpose language suitable for system programming, embedded systems, and other applications where efficiency and performance are crucial.
The syntax of C programming language is derived from the syntax of the B programming language, which was itself based on the syntax of the BCPL programming language. It is a procedural programming language, which means that it is based on the concept of procedures, or routines, which are blocks of code that can be called from other parts of the program.
C programming language is a compiled language, meaning that the code is compiled into machine-readable code by a compiler before it can be executed. This makes it faster and more efficient than interpreted languages, which are executed directly by an interpreter.
C programming language has been the basis for many other programming languages, including C++, Java, Python, and Perl. It is widely used in system programming, operating systems, embedded systems, and other applications where performance and efficiency are important.
In addition to its efficiency and performance, C programming language is also known for its flexibility and portability. Programs written in C can be easily ported to other platforms with minimal modification, making it a popular choice for cross-platform development.
History of C programming language:
C was developed by Dennis Ritchie at Bell Labs in the early 1970s. It was designed as a systems programming language to be used in the Unix operating system. C was created to replace the assembly language used for system programming and to provide low-level access to the computer's hardware.
In 1989, the International Organization for Standardization (ISO) standardized the C language, creating the ANSI C standard. This made it easier for programmers to write portable code that could be compiled on different systems.
It can be specified in the additional ways:
- Mother language
- System programming language
- Procedure-oriented programming language
- Structured programming language
- Mid-level programming language
What is the use of C programming language?
The C language has formed the base of many languages including C++, C-, C#, Objective-C,
BitC, C-shell, csh, D, Java, JavaScript, Go, Rust, Julia, Limbo, LPC, PHP, Python,
Perl, Seed7, Vala, Verilog and many more other languages.
As it is a middle-level language, C combines the features of both high level and low level languages.
So it can be used for low level programming, such as scripting for drivers and kernels.
It also support the functions of high level programming languages, such as scripting for software applications etc. C programming language is evaluated as the base for other programming languages.
That is why it is known as the mother of programming language.
C programming language tutorial with a programming technique for beginners and professionals and it helps
you to know the C programming language tutorial skillfully.
Our C tutorial clarifies each theme with programs.
It can be specified in the additional ways:
- Mother language.
- System programming language.
- Procedure-oriented programming language.
- Structured programming language.
- Mid-level programming language.
1. C as a mother language :
C programming language is assumed as the mother language of all the new programming languages. Because most of the compilers, JVMs, Kernels, etc are written in the C programming language. And most of the programming languages follow C syntax. For example, C++, Java, C#, etc.
It gives the core concepts like the array, strings, functions, file handling, etc. That is being employed in various languages like C++, Java, C#, etc.
2. C programming language as a system programming language :
A system programming language is used to build system software. C programming language is a system programming language because it can be manipulated to do low-level programming. For example, (driver and kernel). It is mostly used to create hardware equipment, OS, drivers, kernels, etc. For example, the Linux kernel is written in the C programming language.
It can't be manipulated for internet programming like Java, .Net, PHP, etc.
3. C as a procedural language:
A procedure language is understood as a function, method, routine, subroutine, etc. A procedural language indicates a series of walks for the program to understand the difficulty.
A procedural language smashes the program into functions, data structures, etc.
C programming language is a procedural language. In C, variables and function prototypes must be announced before being manipulated.
4. C as a structured programming language:
A structured programming language is a subset of procedural speech. Structure means to smash a program into parts or blocks so that it may be easy to discern.
In the C programming language, we break the program into parts using functions. It makes the program simpler to understand and improve.
5. C as a mid-level programming language:
C programming language is considered a middle-level language because it benefits the detail of both low-level and high-level languages. C programming language is converted into assembly code and it supports pointer arithmetic (low-level), but it is device independent (a feature of high-level).
A Low-level language is particular to one machine, i.e., machine-dependent. It is machine-dependent, fast to run. But it is not easy to understand.
A High-Level language is not particular to one machine, i.e., machine-independent. But it is simple to understand.
What are the features of c programming language?
C programming is comprehensively used language over the world. It contains many features which are written below.
- Simple
- Machine Independent or Portable
- Structured programming language
- Mid-level programming language .
- Rich Library.
- Fast Speed
- Memory Management .
- Recursion
- Pointer
- Extensible
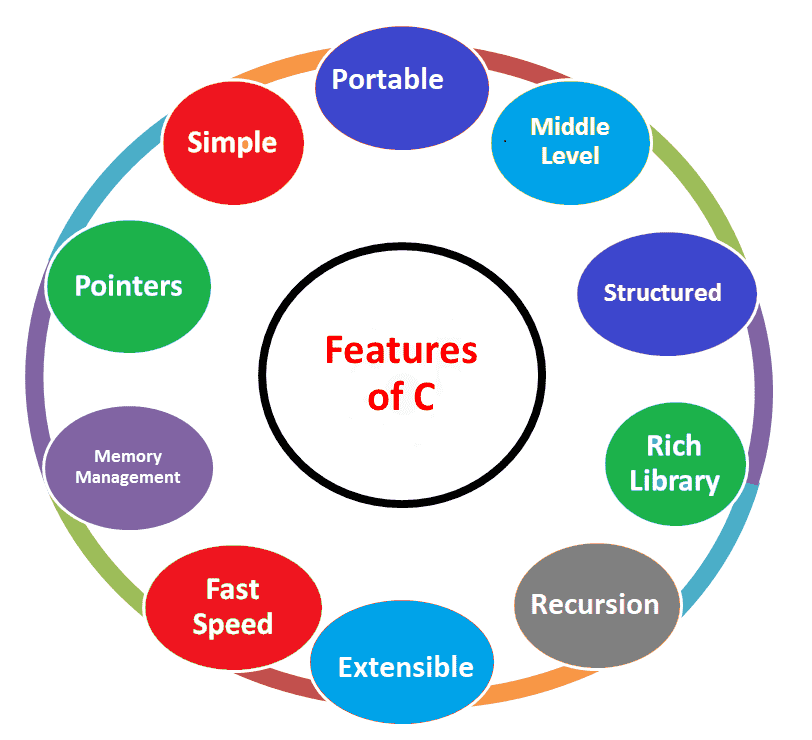
1. Simple:
As a language, C program is a easy language in the mean that it yields a well-structured approach ( to separate the problems in multiple parts), the enriched set of data types, library functions etc.
2. Machine Independent or Portable:
Unalike the assembly language, c program can be performed on various machines altering some specific changes. Thus, we call C program a machine independence language.
3. Structured programming language:
C is a structured programming language in the sense of that we can separate the program into smaller parts by the help of functions. So, it is quite simple to understand and modify. Functions also transmits code reusability.
4. Mid-level programming language:
Though C is executed to do lower level programming, it is also used for development of system applications such as drivers, kernel etc. It also copes up with higher level languages. For this C is called a Mid-level language.
5. Rich Library:
C provides a lot of integral functions that make the development faster.
6. Fast Speed:
The time for compilation and execution of C language is faster than other languages as there are lesser inbuilt functions and thus the lesser overhead.
7. Memory Management:
It supports dynamic memory allocation features . In C , we can set free the allocated memory at any time by calling the free () function.
8. Recursion:
In C, we can call out the function within the function. It yields code reusability for each and every function. Recursion allows us usage the approach of backtracking.
9. Pointers:
C language provides the feature of pointers. We can easily interact with the memory by using the pointers. We can use pointers for arrays ,memories, functions, structures, etc.
10.Extensible:
Since C language can cope up with new features, it is extensible.