Structure of C program
Program strucure of C:
Before we start we need to know about the structure of c program .It will help you to write the codes and you can understand codes very easily. Let's have a look on the basic structure:
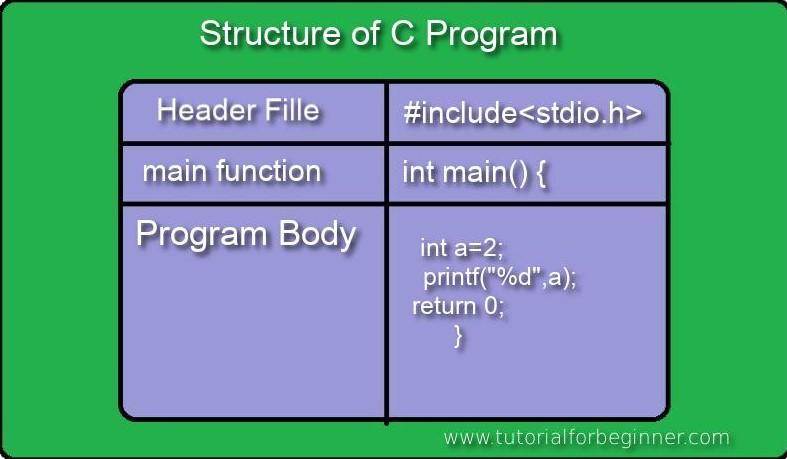
1. Preprocessor Directives:
These are commands that instruct the compiler to do certain tasks before the code is compiled. Examples of preprocessor directives include "#include" to include header files and "#define" to create macros. An example given below:
#include<stdio.h> //Preprocessor Directives
#define pi 3.1416 //Preprocessor Directives
int main()
{
printf("Hello");
return 0;
}
2. Header File:
The first and foremost component is the inclusion of the Header files in a C program top of your program including
.h extension.
May be you are thinking What is the header file? Why should I include it?
Header file is very very important component for c program. You can consider it with human brain.
For an example: Suppose you do not know about cat and never seen before . So it is an unknown animal to you, right? If someone asked you about cats, you would not be able to say anything because there is no information about cats in your brain. Now assume you know about cats. If someone asks you about cats, you will be able to say this only because the information about cats is available in your brain. In the same way, C has some reserved words and functions like
Writing the header file in C:
#include<(header_file_name).h>
Let's familar with some of headerfiles:
# | Header File | Description |
---|---|---|
1 | stdio.h | Basically stdio stands for Standard Input And Output. std for Standard and io for Input and Output. It defines core input and output functions like scanf(), and printf(). |
2 | stdlib.h | It defines numeric conversion functions, pseudo-random network generator and memory allocation like alloc() which is for dynamic memory allocation. |
3 | string.h | It defines string handling functions like strlen() for counting for string length. |
4 | math.h | It defines common mathematical functions like sqrt(), cos() etc which is root function. |
5 | time.h | This header file contains functions for working with time and date, such as time() and localtime(). |
3. Main Function:
This is the function where the program execution begins. It is the primary function of the program and contains the code that performs the main task of the program. An example given below:
#include<stdio.h>
void main() or int main(){
//code here.
}
4. Variables:
These are used to store data and information that is used in the program. They must be declared before they can be used. an example given below:
#include<stdio.h>
int main(){
int add; // variable declaration.
add = 10; // assgn value
printf("%d",a); // print value
return 0;
}
Output:
10
5. Program statements:
These are individual instructions or actions that are performed by the program. Examples of statements include assignments, conditional statements, and loops.
#include<stdio.h>
int main(){//start program body
int a;
a = 5; // statement
printf("Number is: %d",a); // statement
return 0;
}//end program body
Output:
Number is: 5
6. Functions:
These are reusable blocks of code that perform a specific task. They can be called from anywhere in the program and can take parameters as input and return a value as output. an example given below:
#include<stdio.h>
int add(){
printf("I'm from add");
}
int main(){
add(); // calling function
}//end program body
Output:
I'm from add